Implicit vs explicit programming involves some key differences: implicit programming involves the processes happening behind the scenes, while explicit programming is when you clearly state what you want your code to do. Implicit programming is applicable topresent in many coding languages, including C++.
To use the famous “Hello, World!” example, if you write a statement that outputs the text “Hello, World!”, and don’t explicitly state that it should be treated as a string, that’s implicit programming.
We can dig a little deeper and go into the specifics and some more examples.
So What Are Those “Behind The Scenes” Processes?
Implicit programming includes default behaviours, type inference, and built-in functions. Here’s a quick overview:
- Implicit type conversion: Languages like C++ automatically convert one data type to another when needed.
- Implicit constructors: In C++, constructors without the ‘explicit’ keyword can be used for implicit type conversions.
- Implicit return types: Some languages can deduce function return types automatically.
- Type inference: Languages like C++ (with ‘auto’ keyword), Rust, and many functional languages can infer variable types without explicit declarations.
- Operator overloading: Operators can be implicitly called for user-defined types.
- Implicit this pointer: In object-oriented languages, member functions implicitly receive a ‘this’ pointer.
- Default parameters: Functions can have default values for parameters, allowing calls with fewer arguments.
- Implicit interface implementation: Some languages like Go don’t require explicit interface declarations.
Implicit programming can make code more concise and readable, but it requires some understanding to avoid unintended behaviors.
Both implicit programming and end-to-end, or E2E programming can involve abstraction. E2E programming often uses high-level abstractions in complex systems and implicit programming creates abstractions within the language. For more on E2E meaning, check out a comprehensive guide to E2E testing.
Can You Give me Some More Examples of Implicit Programming?
Let’s look at the first process mentioned above: implicit type conversion. C++, for example, can automatically convert one data type into another when it’s appropriate.
An integer may be implicitly converted to a floating-point number. Even though “num”, for example, can be an integer, C++ can implicitly convert it to a floating-point number in the division. This is so the result is accurate (instead of truncating the division result to an integer).
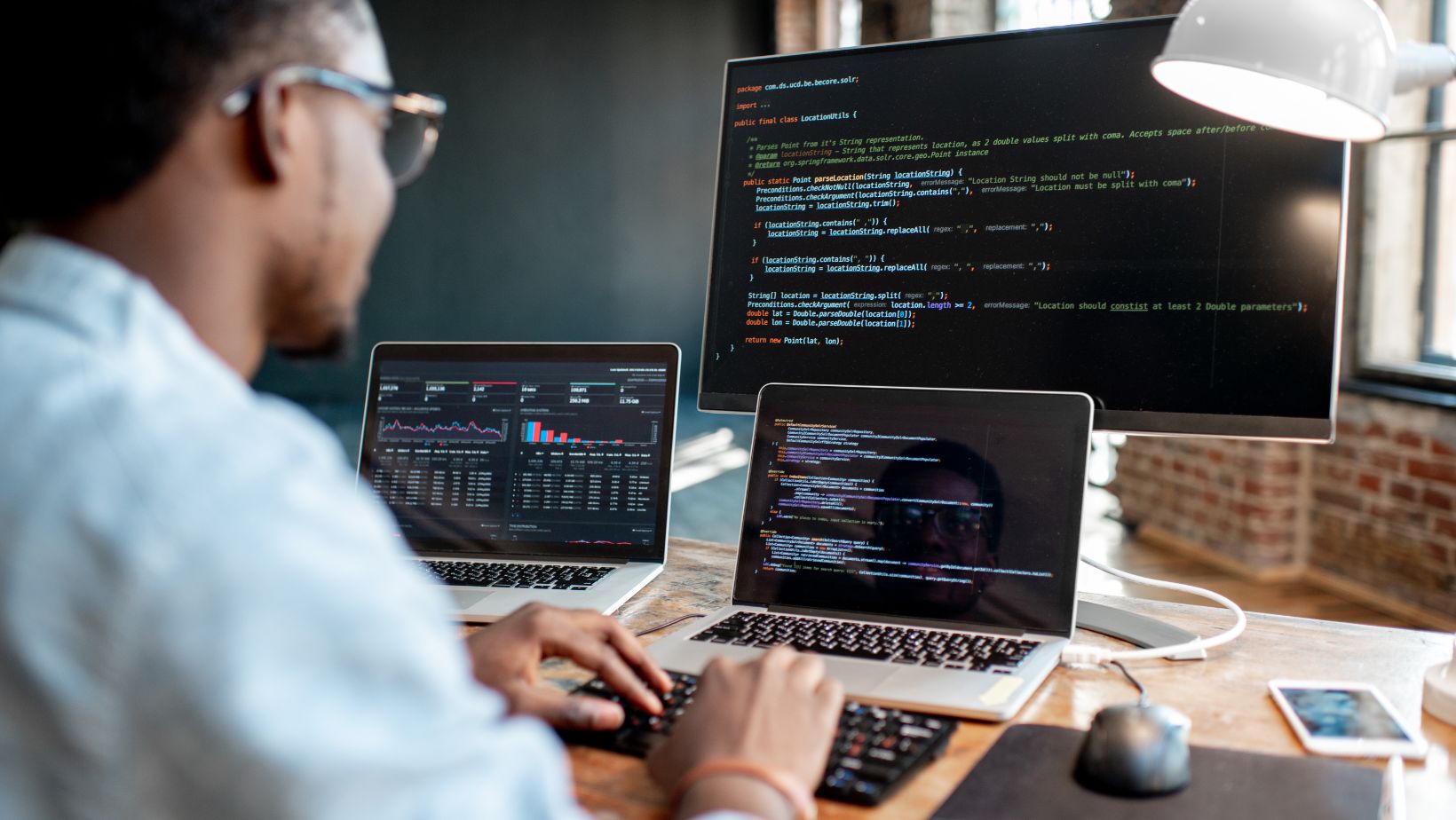
The second process, implicit constructors, may be simpler to understand. Constructors can be called implicitly. For example, the class “Greeting” can automatically print “Hello, World!” when an object is created.
Why is it Important to Know The Difference Between Implicit and Explicit Programming?
Understanding the difference between implicit and explicit programming is important because it impacts how you write, debug, and maintain code. Both of these styles of programming have positives and negatives, and knowing when to use one over the other can help you develop cleaner, more efficient, and less error-prone code.
Which is Used More Often: Implicit or Explicit Code?
This varies depending on the programming language, the context of the application, and your aims as a programmer.
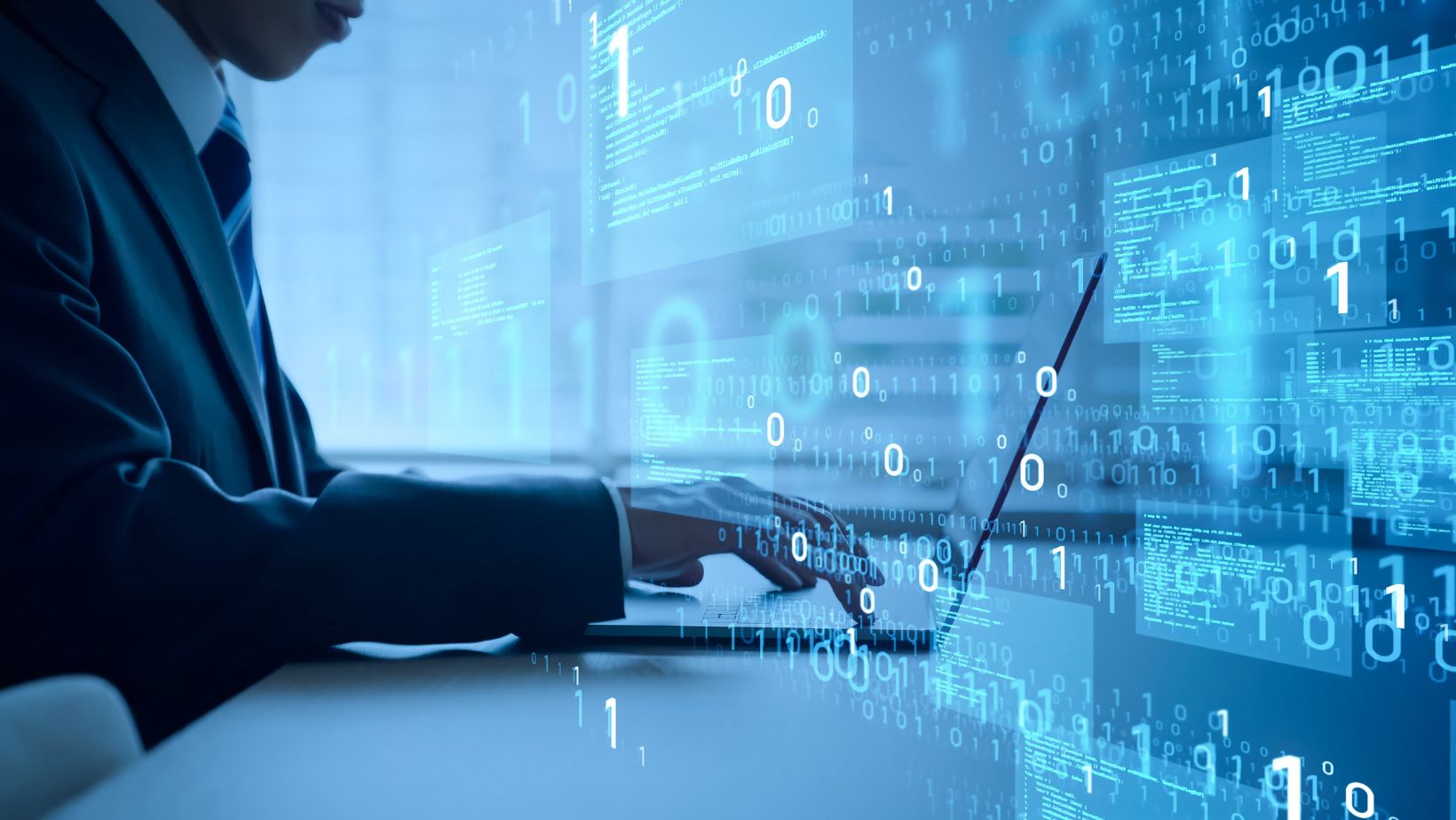
But in general, explicit is used more often in critical, large-scale, and performance-sensitive systems, and implicit code more in situations where simplicity and ease of use are important. By improving your skills in both implicit and explicit programming, you’ll be a well-rounded coder – even if AI does much of the work for us.